Lab 7.2 ConsoleMinesweeper
Reading
If you haven't done so yet, read Java FX Lesson 7 Page B.
Overview
For this HW, you will create a console version of Minesweeper with three classes:
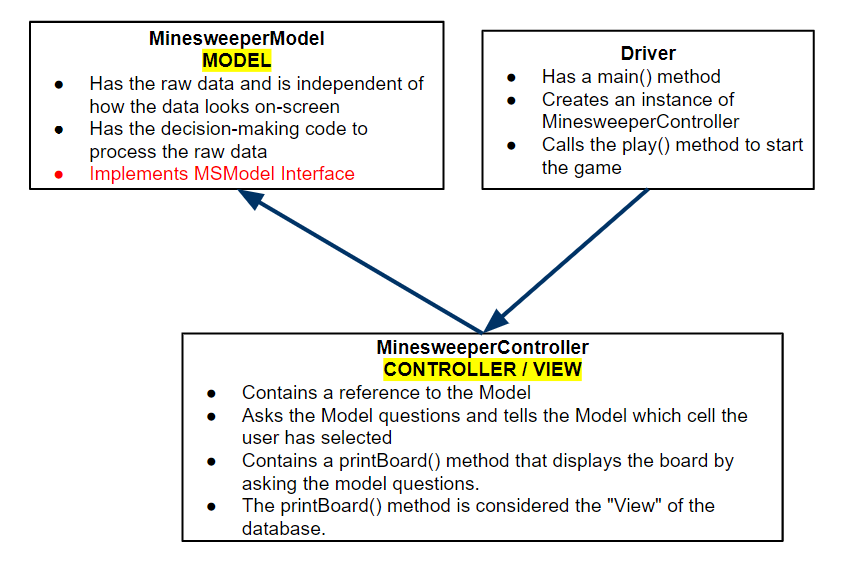
Directions
- Start a new Eclipse project for ConsoleMinesweeper.
- Add the
MSModelInterface
that you came up with.
- Write the
MinesweeperModel
class, which contains the raw data, operations on the data, and implements the MSModelInterface
.
It's up to you how to store the data. Each student will do this differently. Do you want to use arrays, ArrayLists, GridModel, Maps, or something else? What data type will you use in your data structures? Furthermore, since Minesweeper has two layers (cover tiles and tiles below the covers that get revealed) do you want to use parallel 2D arrays for the different layers or one 2D array that holds some kind of object that knows whether it has been revealed? Both styles can work.
After your model is written, create a console version of Minesweeper that uses your model.
- Create a new Java class named
PX_LastName_FirstName_MinesweeperController
.
- Add a constructor that accepts the number of rows, columns, and total number of mines.
- For this program, the "view" will just be a printBoard() method in the
PX_LastName_FirstName_MinesweeperController
class. Your printBoard() should ask the model questions and then choose how to display that information.
- Add a play() method that handles the main game loop. This method should do something like:
- Print an intro messages and print the initial board (helper method)
- Enter a loop that repeatedly does:
- Ask the user whether they want to flag 'f or reveal 'r'
- Ask the user for the row, col they want to select
- If flag, tell the model to flag that location
- If reveal, tell the model to recursively reveal that location
- Ask the model if the location was a mine. If so, game over.
- Ask the model if all non-mine cells have been revealed. If so, you win.
- Print the board
- Repeat the loop while game is not over or won.
- The player's first move should never be a mine. To accomplish this, you have two options:
- Generate the minefield first, but if the user's first move happens to be a mine, move that mine to a random non-mine location
- Or, generate the minefield after the user's first move.
- Display messages when any of the following happen:
- Player reveals a mine → You Lose
- Player reveals all non-mines → You Win
- After each move you must print out (for debugging purposes)
- The lower layer (that shows mine locations, numbered cells, and blank cells)
- The upper layer (that shows what the player sees)
- The number of mines remaining (This is really the total number of mines minus the number of flags. If there were 10 mines and 12 flags placed, you would display -2)
Here is example output for this program.
Click here for testing checklist.
Files to submit
PX_LastName_FirstName_MSModelInterface.java
PX_LastName_FirstName_MinesweeperController.java
PX_LastName_FirstName_MinesweeperModel.java
PX_LastName_FirstName_MinesweeperDriver.java
You must Sign In to submit to this assignment